Did you know connecting a database in PHP with MySQL on XAMPP takes only 3 lines of code? This shows how powerful today’s web development tools are. They make it easy for developers to link their PHP apps to strong MySQL databases. We’ll explore the basics of XAMPP and MySQL, focusing on how to make secure and fast database connections in your PHP projects.
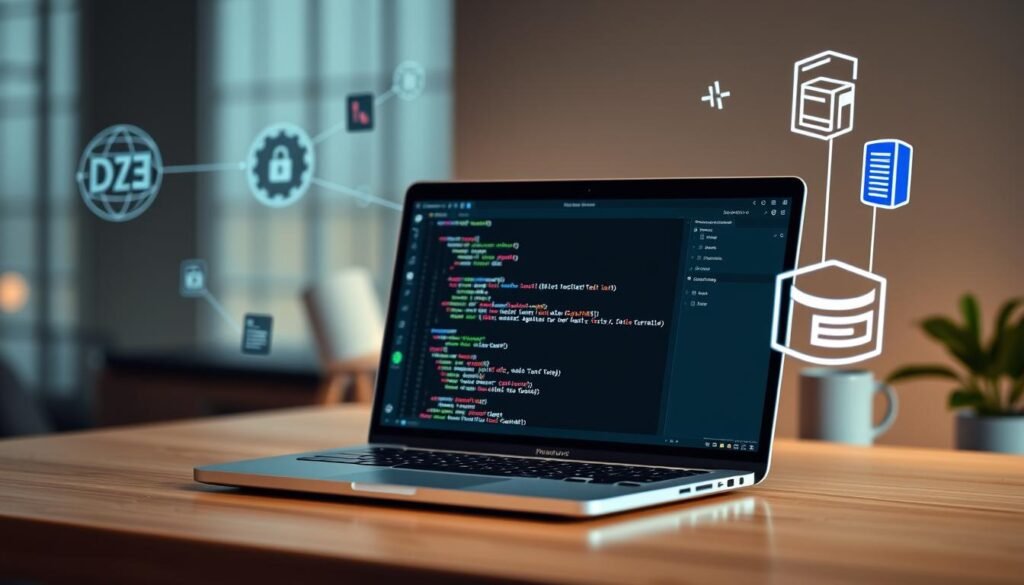
Key Takeaways
- Connecting to a MySQL database in PHP on XAMPP requires just 3 lines of code.
- Socket connections are the preferred method for connecting to MySQL/MariaDB for performance reasons.
- PDO can work with 12 different database systems, while MySQLi is specific to MySQL.
- Both PDO and MySQLi support Prepared Statements to prevent SQL injection attacks.
- The MySQLi extension is often pre-installed when the PHP5 mysql package is installed.
Understanding XAMPP and MySQL Database Fundamentals
XAMPP is a free software package that makes setting up a local web server easy. It has Apache, MySQL, and PHP. Developers can test their PHP apps on their computers before they go live.
What is XAMPP and Its Components
XAMPP stands for “Cross-Platform, Apache, MySQL, PHP, and Perl”. It’s a full web development environment. The main parts of XAMPP are:
- Apache – The web server that handles HTTP requests and delivers web content.
- MySQL – The database system that stores and manages data for web apps.
- PHP – The scripting language for dynamic web pages and apps.
- Perl – An extra programming language in XAMPP.
MySQL Server Configuration in XAMPP
The MySQL server in XAMPP uses port 3306 by default. To connect, you need the right username and password. Usually, the default username is “root” and the password is also “root”.
Essential Prerequisites for Database Connection
To connect to a database in your PHP app, you need to:
- Set up XAMPP correctly on your machine.
- Make sure the MySQL server is running and accessible through the XAMPP Control Panel.
- Get the right database credentials, like username and password, to connect to MySQL.
Knowing about XAMPP and how to set up MySQL will help you make secure database connections in your PHP apps.
Database connection in php with mysql in xampp code example
If you’re a PHP developer, you’re in the right spot. We’ll show you how to connect your web apps to a MySQL database. We’ll use the XAMPP development environment for this.
XAMPP is a great tool for local web development. It comes with Apache, MySQL, and PHP. Make sure you have XAMPP installed and MySQL running.
Here’s a simple PHP script for connecting to a MySQL database using mysqli
:
connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
Now, let’s explain the code:
- We set the server name, username, and password for the MySQL connection.
- We create a new
mysqli
connection object with the given credentials. - We check if the connection was successful by looking at the
connect_error
property. - If it fails, we display the error message and stop the script with
die()
. - If it works, we just echo “Connected successfully”.
This example shows how to connect to a MySQL database with PHP and mysqli
. Next, we’ll explore more advanced ways to connect securely and handle errors.
Got questions about php code examples, database connectivity, or mysqli functions? Join our PHP community on Facebook. Use the #PHPMySQL
hashtag to ask your questions.
Establishing Secure Database Connections Using MySQLi
PHP uses the MySQLi extension for secure database connections. It offers two ways: object-oriented and procedural. Your choice depends on your coding style and project needs.
Object-Oriented vs. Procedural Approaches
The object-oriented method is more intuitive and organized. It uses the MySQLi class for database operations. The procedural method, on the other hand, relies on standalone functions like mysqli_connect().
Implementing Connection Security Best Practices
It’s vital to secure your database connections, no matter the method. Using prepared statements is a top way to prevent SQL injection attacks. They keep SQL queries separate from user input, stopping malicious code.
Handling Connection Errors and Exceptions
Dealing with connection errors is crucial. MySQLi’s mysqli_connect_error()
function helps get error messages. You can show a friendly error message or log it for later.
Knowing how to secure database connections with MySQLi makes your PHP apps safe and efficient. This is key when integrating with a MySQL database.
Approach | Advantages | Disadvantages |
---|---|---|
Object-Oriented | More organized and intuitive code structure Easier to manage and maintain multiple database connections Supports modern object-oriented programming practices | Slightly more verbose syntax compared to procedural May have a slight performance overhead due to the additional object-oriented layer |
Procedural | Simpler and more concise syntax May have slightly better performance compared to object-oriented Easier to integrate with existing procedural code | Less organized and harder to manage multiple database connections Doesn’t take advantage of modern object-oriented programming practices |
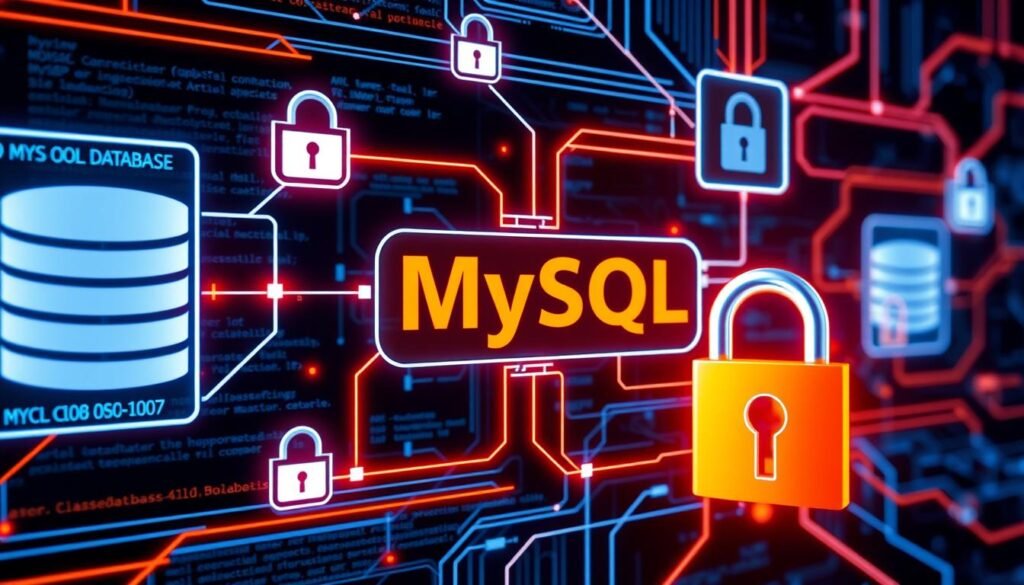
Advanced MySQL Integration Techniques in PHP
As a PHP developer, learning advanced MySQL techniques can boost your app’s performance and security. We’ve covered the basics, now let’s explore powerful tools and strategies. These can elevate your PHP-MySQL projects.
PHP Data Objects (PDO) is a versatile solution. It connects to various databases, including MySQL, PostgreSQL, and SQLite. Unlike MySQLi, which is only for MySQL, PDO works with different databases. This makes it easier to switch databases later.
Connection pooling is another advanced technique. It keeps a pool of open connections ready. This boosts performance, especially in busy apps, by cutting down on connection creation and closure overhead.
Using transactions is key for keeping data safe in MySQL apps. Transactions make sure all database changes are either fully applied or rolled back. This prevents partial updates and keeps data consistent.
To wrap up, advanced MySQL techniques in PHP include:
- Using PDO for flexible database connections
- Setting up connection pooling for better performance
- Managing transactions for data integrity
- Choosing the right character set (like utf8mb4) for handling special characters
By mastering these techniques, you can make your PHP-MySQL apps more robust and scalable. They’ll be ready for the future needs of your users.
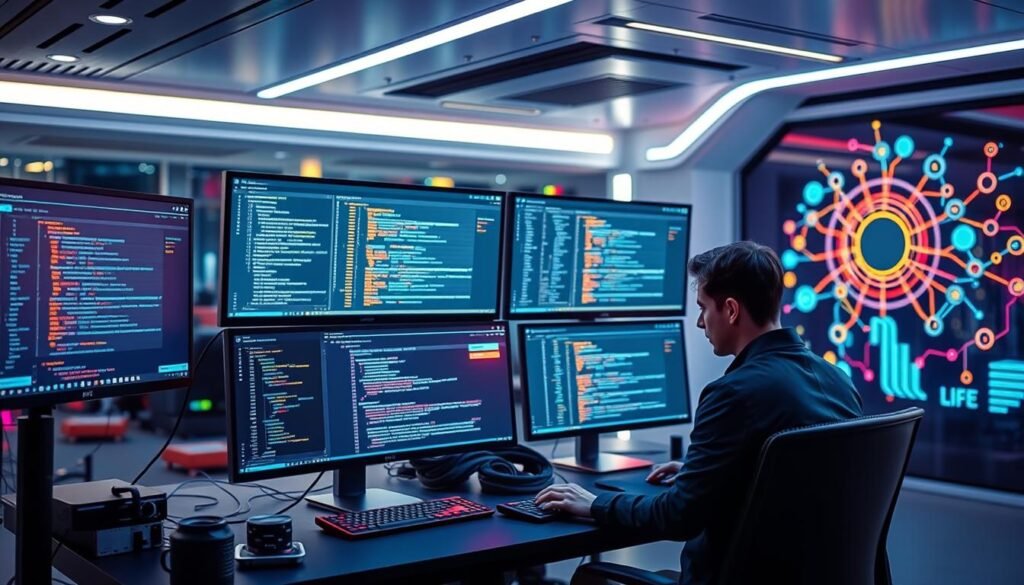
Conclusion
In this article, you’ve learned the basics of connecting a database in PHP with MySQL using XAMPP. You now know about XAMPP’s key parts, how to set up the MySQL server, and what you need for a successful database connection.
You can use either the MySQLi object-oriented method or the simpler procedural way. You have the knowledge to make secure and strong database connections in your PHP projects. Always follow best practices, handle errors well, and test your database connection often for a smooth user experience.
With what you’ve learned, you’re getting better at connecting databases in PHP with MySQL in XAMPP. Keep practicing, trying new things, and keep up with PHP and database management news. This will help you get even better at this important part of web development.
FAQ
What is the process for establishing a database connection in PHP with MySQL in XAMPP?
To connect a database in PHP with MySQL in XAMPP, start by creating a file in the htdocs folder. You’ll need to enter the server name, username, and password. Make sure to use the right port number, like 3306 for MySQL.
Using a socket connection is better than TCP/IP for MySQL/MariaDB. This can improve your performance.
What is XAMPP and what are its components?
XAMPP is a bundle that includes Apache, MySQL, PHP, and Perl. MySQL in XAMPP runs on port 3306. To connect, you need to set up XAMPP correctly and have the right credentials.
The default username is often “root” with no password or “root” as the password. This is important for connecting.
Can you provide a basic PHP code example for MySQL connection in XAMPP?
Here’s a simple PHP code for connecting to MySQL in XAMPP: php connect_error) { die(“Connection failed: ” . $conn->connect_error); } echo “Connected successfully”; ?> This code connects to the MySQL server and checks for errors.
What are the different approaches for database connections in PHP?
MySQLi has two ways to connect: object-oriented and procedural. The object-oriented method uses the mysqli class. The procedural method uses mysqli_connect().
For secure connections, use prepared statements to avoid SQL injection. You can handle errors with try-catch blocks in PDO or by checking for connection errors in MySQLi.
What are some advanced techniques for MySQL integration in PHP?
Advanced techniques include using PDO for database-agnostic connections and implementing connection pooling for better performance. PDO supports 12 databases and makes migration easy.
It’s also good to set the character set, like utf8mb4, for proper encoding of special characters.
Also Read